POSCAR2findsymm
March 24, 2009 Posted by Emre S. Tasci
A very simple code I wrote while studying the Python language that fetches the information from a VASP POSCAR file and prepares (and feeds) the input for Harold Stokes’ findsym (you should actually download the executable included in the ISOTROPY package instead of the referred web version) program to find the unit cell symmetry.
#!/usr/bin/python
# -*- coding: utf-8 -*-
"""
POSCAR2findsym.py
/* Emre S. Tasci <e.tasci@tudelft.nl> *
A simple python code that parses the POSCAR file,
prepares an input file for Harold Stokes' findsym
(http://stokes.byu.edu/isotropy.html)
executable.
Then, checks the current system to see if findsym is
accessible: if it is, then feeds the prepared file
and directly outputs the result; if findsym is not
executable then outputs the generated input file.
If you are interested only in the results section of
the findsymm output, you should append "| grep "\-\-\-\-\-\-" -A1000"
to the execution of this code.
Accepts alternative POSCAR filename for argument
(default = POSCAR)
If a second argument is proposed then acts as if
findsym is not accessible (ie, prints out the input
file instead of feeding it to findsymm)
10/03/09 */
"""
import sys
import re
from numpy import *
from os import popen
import commands
force_output = False
if len(sys.argv)<2:
filename="POSCAR"
elif len(sys.argv)<3:
filename=sys.argv[1]
else:
filename=sys.argv[1]
force_output = True
f = open(filename,'r')
title = f.readline().strip()
tolerance = 0.00001
latt_vec_type = 1 # We will be specifying in vectors
f.readline() # Read Dummy
lat_vec = array([])
for i in range(0,3):
lat_vec = append(lat_vec,f.readline().strip())
# Read atom species
species_count = f.readline()
species_count = re.split('[\ ]+', species_count.strip())
species_count = map(int,species_count)
number_of_species = len(species_count)
total_atoms = sum(species_count)
initial_guess = "P" # For the centering
species_designation = array([])
for i in range(1,number_of_species+1):
for j in range(0,species_count[i-1]):
species_designation = append(species_designation,i)
species_designation = map(str,map(int,species_designation))
# print species_designation
sep = " "
# print sep.join(species_designation)
# Read Coordinates
f.readline() # Read Dummy
pos_vec = array([])
for i in range(0,total_atoms):
pos_vec = append(pos_vec,f.readline().strip())
# print pos_vec
findsym_input = array([])
findsym_input = append(findsym_input, title)
findsym_input = append(findsym_input, tolerance)
findsym_input = append(findsym_input, latt_vec_type)
findsym_input = append(findsym_input, lat_vec)
findsym_input = append(findsym_input, 2)
findsym_input = append(findsym_input, initial_guess)
findsym_input = append(findsym_input, total_atoms)
findsym_input = append(findsym_input, species_designation)
findsym_input = append(findsym_input, pos_vec)
# print findsym_input
sep = "\n"
findsym_input_txt = sep.join(findsym_input)
# print findsym_input_txt
# Check if findsym is accessible:
status,output = commands.getstatusoutput("echo \"\n\n\"|findsym ")
if(output.find("command not found")!=-1 or force_output):
# if it is not there, then just output the input
print findsym_input_txt
elif(status==6144):
# feed it to findsym
pipe = popen("echo \""+findsym_input_txt+"\" | findsym").readlines()
for line in pipe:
print line.strip()
quit()
Example Usage:
sururi@husniya POSCAR2findsym $ cat POSCAR
Si(S)-Au(Pd) -- Pd3S
1.00000000000000
4.7454403619558345 0.0098182468538853 0.0000000000000000
-1.4895902658503473 4.5055989020479856 0.0000000000000000
0.0000000000000000 0.0000000000000000 7.2191545483192190
6 2
Direct
0.1330548697855782 0.5102695954022698 0.2500000000000000
0.4897304045977232 0.8669451452144267 0.7500000000000000
0.5128136657304309 0.1302873334247993 0.2500000000000000
0.8697126665752007 0.4871863042695738 0.7500000000000000
0.0013210250693640 0.9986789749306360 0.0000000000000000
0.0013210250693640 0.9986789749306360 0.5000000000000000
0.6856021298170862 0.6825558526447357 0.2500000000000000
0.3174442073552622 0.3143978111829124 0.7500000000000000
sururi@husniya POSCAR2findsym $ python POSCAR2findsym.py
FINDSYM, Version 3.2.3, August 2007
Written by Harold T. Stokes and Dorian M. Hatch
Brigham Young University
Si(S)-Au(Pd) -- Pd3S
Tolerance: 0.00001
Lattice vectors in cartesian coordinates:
4.74544 0.00982 0.00000
-1.48959 4.50560 0.00000
0.00000 0.00000 7.21915
Lattice parameters, a,b,c,alpha,beta,gamma:
4.74545 4.74545 7.21915 90.00000 90.00000 108.17579
Centering: P
Number of atoms in unit cell:
8
Type of each atom:
1 1 1 1 1 1 2 2
Position of each atom (dimensionless coordinates)
1 0.13305 0.51027 0.25000
2 0.48973 0.86695 0.75000
3 0.51281 0.13029 0.25000
4 0.86971 0.48719 0.75000
5 0.00132 0.99868 0.00000
6 0.00132 0.99868 0.50000
7 0.68560 0.68256 0.25000
8 0.31744 0.31440 0.75000
------------------------------------------
Space Group 40 C2v-16 Ama2
Origin at 0.00000 0.00000 0.50000
Vectors a,b,c:
0.00000 0.00000 1.00000
-1.00000 -1.00000 0.00000
1.00000 -1.00000 0.00000
Values of a,b,c,alpha,beta,gamma:
7.21915 5.56683 7.68685 90.00000 90.00000 90.00000
Atomic positions in terms of a,b,c:
Wyckoff position b, y = -0.17834, z = 0.31139
1 0.75000 0.17834 0.31139
2 0.25000 0.82166 0.31139
Wyckoff position b, y = 0.32155, z = 0.19126
3 0.75000 0.17845 0.69126
4 0.25000 0.82155 0.69126
Wyckoff position a, z = 0.00132
5 0.50000 0.00000 0.00132
6 0.00000 0.00000 0.00132
Wyckoff position b, y = -0.31592, z = 0.00152
7 0.75000 0.81592 0.50152
8 0.25000 0.18408 0.50152
Update on the things about the scientific me since October..
March 23, 2009 Posted by Emre S. Tasci
Now that I’ve started to once again updating this scientific blog of mine, let me also talk about the things that occured since the last entry on October (or even going before that).
Meeting with Dr. Villars
The biggest event that occured to me was meeting with Dr. Pierre Villars, the person behind the Pauling File database which I have benefitted and still benefitting from. When I had registered to the “MITS 2008 – International Symposium on Materials Database”, an event hosted by the NIMS Materials Database, I had no idea that Dr. Villars would also be attending to the event, so it was a real surprise learning afterwards that I’d have a chance to meet him in person.
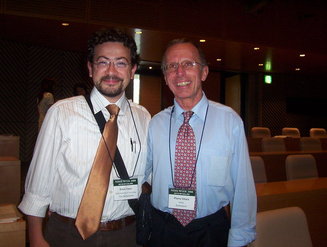
You can read the sheer happiness from my face on the occasion of meeting Dr. Villars
Dr. Villars is a very kind and a cheerful person, I had a greatest time knowing him and afterwards I had the privilege of visiting him in his home office in the lovely town of Vitznau, Switzerland where I enjoyed the excellent hospitality of the Villars family. Dr. Villars helped me generously in providing the data in my research project for finding transformation toughening materials through datamining which I’m indebted for.
The MITS 2008 – Int’l Symposium on Materials Database
I was frequently using the databases on the NIMS’ website and had the honour to participate in the last year’s event together with my dear supervisor Dr. Marcel Sluiter. On that occasion, I presented two talks – a materials based one, and another about database query&retrieval methods- titled “Inference from Various Material Properties Using Mutual Information and Clustering Methods” and “Proposal of the XML Specification as a Standard for Materials Database Query and Result Retrieval”, respectively.
I have an ever growing interest on the Japanese Culture since I was a youngster and due to this interest, my expectations from this trip to Japan was high enough for me to be afraid of the fact that I may have disappointed since it was next-to-impossible for anything to fulfill the expectations I had built upon. But, amazingly, I was proved false: the experience I had in Japan were far more superior to anything I had anticipated. The hospitality of the organizing commitee was incredible: everything had been taken care of and everyone was as kind as one could be. I had the chance to meet Dr. Villars as I’ve already wrote about above, and also Dr. Xu, Dr. Vanderah, Dr. Dayal and Dr. Sims and had a great time. It was also due to this meeting, I met a dear friend.
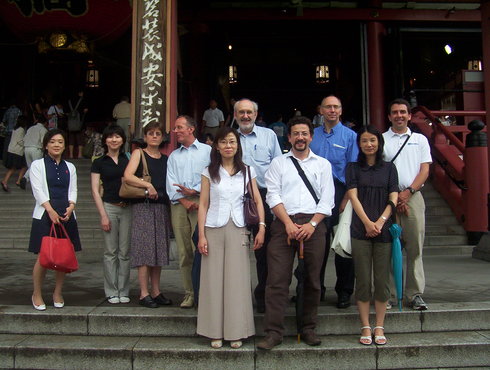
Ongoing Projects
During my time here, I still don’t have anything published and that’s the number one issue that’s bothering me. Apart from my research on the transformation toughening materials, I worked for a time on a question regarding to a related phenomenon, namely about the martensitic transformation mechanism on some transition-metal alloy types. We were able to come up with a nice equation and adequate coefficients that caught the calculation results but couldn’t manage to transfer it to -so to say- a nicely put theory and that doomed the research to being an “ad-hoc” approach. I’ll be returning to it at the first chance I get, so currently, it’s been put on ice (and now remembering how, in the heat of the research I was afraid that someone else would come up with the formula and it would be too late for me.. sad, so sad..). One other (side) project was related to approximate a specific amorphous state in regards with some basis structures obtained using datamining. This project was really exciting and fun to work on because I got to use different methods together: first some datamining, then DFT calculations on the obtained structures and finally thermodynamics to extrapolate to higher temperatures. We’re at the stage of revising the draft at the moment (gratefully!).
Life in the Netherlands & at the TUDelft
It has been almost one and a half year since I started my postdoc here in TUDelft. When we first came here, my daughter was still hadn’t started speaking (yet she had been practicing walking) and now she approximately spent the same amount of time in the Netherlands as she had spent in Turkey (and thankfully, she can speak and also running to everywhere! 8).
When I had come here, I was a nano-physicist, experienced in MD simulations and -independently- a coder when the need arouse. Now, I’m more of a materials guy and coding for the purpose.. 8) I enjoy the wide variety of the jobs & disciplines people around me are working on and definitely benefitting from the works of my friends in our group. I couldn’t ask for more, really. (OK, maybe I’d agree if somebody would come up with an idea for less windy and more sunny weather here in Delft. That’s the one thing I couldn’t still get used to. Delft is a marvelous city with bikes and kind people everywhere, canals full of lillies in the spring, historical buildings everywhere, Amsterdam, Den Haag and Rotterdam just a couple of train stations away and if you wish, Antwerpen, Brussels and Brugges (Belgium) is your next-door neighbour but this dark and closed weather can sometimes be a little mood lowering.) TUDelft is really a wonderful place to be with all the resources and connections that makes you feel you can learn anything and meet anyone that is relevant to the work you’re doing without worrying about the cost. And you’re being kept informed about the events taking place about you through the frequent meetings in your group, in your section, in your department, in your field. I’ve learned a lot and I’m really happy I was accepted here.
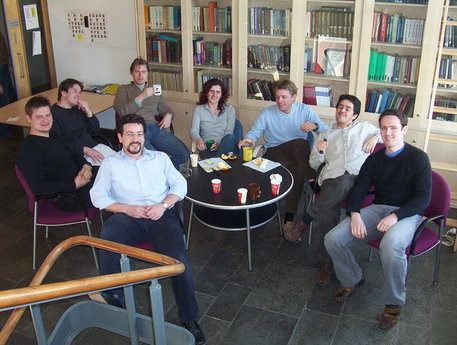
So that’s about the things I’ve enjoyed experiencing and living through.. And I promise, I’ll be posting more regularly and frequently from now on..
Yours the chatty.
LaTeX to PNG and all that equations..
Posted by Emre S. Tasci
After migrating this blog to a new server, I had some difficulties in writing up the equations. It was due to the fact that on this server, I wasn’t able to execute the necessary commands to parse the latex code and convert it to PNG format.
Last week, some need arouse for this and I finally coded the necessary way around to be able to convert LaTeX definitions to their PNG counterparts. Before I start to quote the necessary code and explanations, let me tell that, this method only will work if you can successfully manage to produce this conversation at your -local- computer since what the code does is to generate the image on your local computer and then copy it to the server where your blog (/or whatever you have) resides. So, make sure:
- You can convert LaTeX code to PNG on your -own- computer.
- You are running a httpd server so that the code can copy the output PNG file from your local computer
What the code does:
It has 3 seperate processes. Let’s designate them A, B & C.
- Part A is called on the remote server. It presents a form with textbox in it where you can type the LaTeX code. The form’s target is the same file but this time on your local server, so when you submit the data —
- Part B is called on your local server. Using the texvc code, an open source software that also comes packed with mediawiki, it converts the LaTeX code into a PNG image and places it under the relative math/ directory of your local server. After doing this, via the META tag ‘refresh’ it causes the page to refresh itself loading its correspondence in the remote server, hence initiating —
- Part C where the generated image identified by the MD5 hashname is fetched from the local server and copied unto the relative math/ directory on the remote server. The LaTeX code that was used is also passed in the GET method and it is included in the generated code as the title of the image, so if necessary, the equation can be modified in the future.
The code is as follows:
<?PHP if($_GET["com"]||(!$_POST["formula"] && !$_GET["file"])){?>
<body onload="document.postform.formula.focus();">
<form action="http://yourlocalserver.dns.name/tex/index.php" method="post" name=postform id=postform>
<textarea name="formula" cols=40 rows=5 id=formula>
</textarea><br>
<input type="submit" value="Submit" />
<?PHP
}
if($_POST["formula"])
{
// NL
$lol = './texvc ./mathtemp ./math "'.$_POST["formula"].'" iso-8859-1';
exec($lol,$out);
$file = substr($out[0],1,32);
$formula = base64_encode($_POST["formula"]);
$html = '<HTML>
<HEAD>
<META http-equiv="refresh" content="0;URL=http://remoteserver.dns.name/tex/index.php?formula='.$formula.'&file='.$file.'">
</HEAD>';
echo $html;
}
else if($_GET["file"])
{
// COM
$file = "math/".$_GET["file"].".png";
if(!copy("yourlocalserver.dns.name/tex/".$file, $file)) exit( "copy failed<br>");
$formula = base64_decode($_GET["formula"]);
?>
<form action="http://yourlocalserver.dns.name/tex/index.php" method="post">
<textarea name="formula" cols=40 rows=5><?PHP echo $formula; ?>
</textarea><br>
<input type="submit" value="Submit" />
<?
echo "<br><br><img src=\"".$file."\" title=\"".$formula."\"><br>";
echo "<img src=\"/tex/".$file."\" title=\"".$formula."\"><br>";
}
?>
To apply this:
1. Create a directory called ‘tex’ on both servers.
2. Create subdir ‘math’ on both servers
3. Create subdir ‘mathtemp’ on the local server
4. Modify the permissions of these subdirs accordingly
5. Place the executable ‘texvc’ in the ‘tex’ directory
6. Place the code quoted above in the ‘tex’ directories of both servers in a file called ‘index.php’
If everything well up to this point, let’s give it a try and you shall have some output similar to this:
For an input LaTeX code of:
\sum_{n=0}^\infty\frac{x^n}{2n!}
where you can immediately see the resulting image, modify the code if there’s something wrong or copy the IMG tag and put it to your raw HTML input box in your text…